New in Latte 2.6: optional chaining and custom functions
Latte 2.6 was recently released, which comes with two smart innovations that will simplify and streamline your templates. Let's look at them.
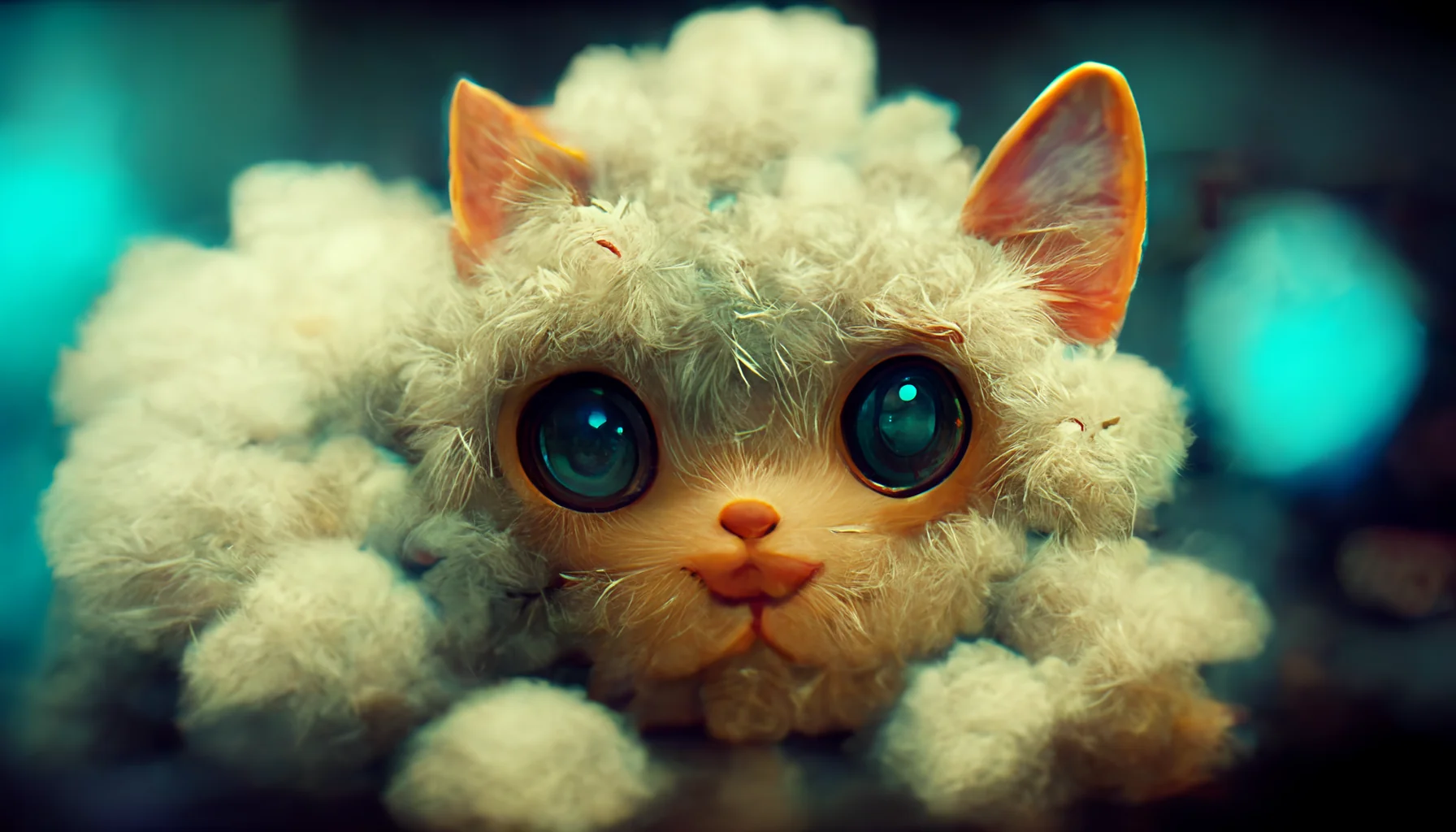
Optional Chaining
Optional chaining lets us write code where Latte immediately stops evaluating
expressions if it encounters null. With the new ?
operator allowing
optional access to variables or array elements. When we write code like
{$order?->id}
this is a way of saying that when $order
is defined,
$order->id
will be computed, but when $order
is
null, stop what we’re doing and just return null.
You might find yourself using ?
to replace a lot of code that
performs repetitive nullish checks:
{$user?->address?->street}
// roughly means isset($user) && isset($user->address) ? $user->address->street : null
{$items[2]?->count}
// roughly means isset($items[2]) ? $items[2]->count : null
{$user->getIdentity()?->name}
// roughly means $user->getIdentity() !== null ? $user->getIdentity()->name : null
In the examples I write “roughly” because in fact the expression is
evaluated more sophistically and no step is repeated. For example,
$user->getIdentity()
is called only once, so therefore the
problem cannot occur because the method returns an object for the first time and
null for the second time.
Optional chaining expressions can be used anywhere, for example in the conditions:
{if $blogPost?->count('*')}
// means if (isset($blogPost) && $blogPost->count('*'))
...
{/if}
Custom Functions
You can use all the global functions of PHP, but now also define your own:
$latte = new Latte\Engine;
$latte->addFunction('random', function (...$args) {
return $args[array_rand($args)];
});
Or in presenter (requires nette/application v3.0.3):
$this->template->addFunction('random', function (...$args) {
return array_rand($args);
});
The usage is then the same as when calling the PHP function:
{random(apple, orange, lemon)} // prints for example: apple
Support for custom features can be found in the new version of the Latte plugin for PhpStorm (so far in the beta version here).
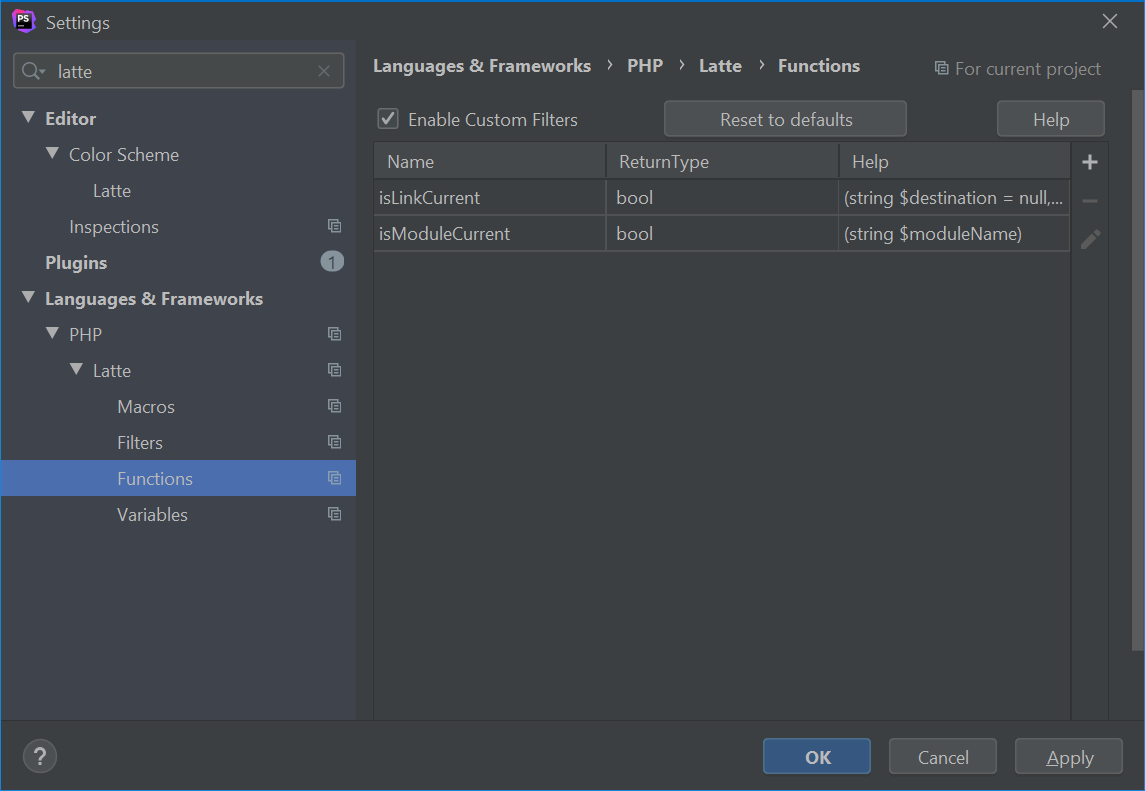
As the picture suggests, a pair of isLinkCurrent()
and
isModuleCurrent()
functions will automatically be available in the
template along with the new version of nette/application
.
<a n:href="Photo:" n:class="isLinkCurrent('Photo:') ? actual">Photogallery</a>
Sign in to submit a comment